How to get started with PHP Jet
1. Installation
If you just want to get a feel for PHP Jet, then the procedure is very simple:
- Make sure you have PHP 8 installed, including all common modules INTL, PDO and mbstring.
-
Download the package from GitHub and unzip it.
or
You can use composer:composer create-project mirekmarek/php-jet
- Go to the directory where you have downloaded and extracted PHP Jet.
- Start the playground:
php _playground/start.php
- Open the URL http://localhost:8000/ in your browser (unless you specified a different address and port when you started the playground).
- If you want, then you can create some test database on MariaDB/MySQL, PostgreSQL and MS SQL (and Oracle in the near future), but it is not necessary. PHP Jet also supports SQLite and that is enough for testing purpose.
- Finish the installation using the web installer.
Running on a full-fledged web server (Apache, NGINx) is also easy. See documentation.
2. Quick introduction to the sample application
After you "click through" the installer (which, by the way, is the general basis of an installer, on the basis of which you can create your installer - for your software product), then you will see the whole sample application, which includes:
- Simple website containing many subpages, which are mostly a demonstration of standard PHP Jet capabilities and also a test of various features, whether it is localizability, forms, ORM, or REST server and client and so on.
- A non-public part of the site to demonstrate and test the authentication and authorization capabilities, including linking to Jet MVC.
- Administration with a number of modules (e.g. for user and role management, viewing logs, but also the primitive basis of the CMS).
- REST API server and client for testing.
- Very helpful development environment Jet Studio with web graphical UI.
- Integrated profiler.
- ... And all this divided into application modules (microapplications) to keep the project in order. The sample application takes full advantage of the unique philosophy of Jet MVC, of course, ORM DataModel, and a host of other capabilities of the PHP Jet framework.
Everything is set up so that you can explore, test, change, just "play". Right away you have the basis of a real application neatly divided into clear structures.
3. The first "route"
PHP Jet doesn't have any routs in the sense you know from other frameworks, but it has something much better.
How the Jet MVC works is a topic in itself, but I'll try to explain it as briefly as possible here.
The basic idea is that every web application consists of bases and pages. The individual pages are made up of a layout. Individual application modules are then run within the pages - as micro-applications. And it is only within these micro-applications that you will find something that is similar to the routing you may be familiar with from other frameworks.
Base determines what part of the application it is (Is it the administration? Is it the API? Is it anything else?). A base is an entity to work with. The Base also supports localizability - supporting multiple national versions of the application.
A page is also an entity to work with and bind content to.It is possible to create navigation between pages, change their layout and URL while maintaining the functionality of the whole project.
Application modules are located within the page and have their own routing.
Everything is defined only by the definitions stored in the files. Nothing uses the database. The whole system is very fast and uncomplicated, optimized for very large projects indeed.
Best of all, you can create the entire structure of your application (for example, a website) conveniently using Jet Studio.
4. Localizability
You may have noticed that localizability is an integral part of PHP Jet. Already Jet MVC firmly counts on application localizability. And thus, of course, the translator or formatting information according to national conventions must not be missed.
5. Application modules / micro-applications
And the recurring theme of application modules has not escaped you. There has been a lot of talk lately about micro-services architecture. Know that this is actually the same thing. It's the same philosophy and the same benefits - breaking down a project into small, easily maintainable and ideally reusable micro-applications. That's PHP Jet itself - it was conceived and designed that way right from the start.
6. ORM
Integrated ORM DataModel is typical in that it's really an ORM. That is, it looks at data entities not from the perspective of data tables in a relational database and SQL, but from the perspective of classes. When you design (click in Jet Studio - why do it manually?) for example an entity representing an article or a product in an e-shop, then you think primarily in terms of classes and their arrangement. The DataModel then takes care of such "annoying" things as saving, loading, updating and deleting data.
Look no further than the migrations directory. Entity definitions (how they are stored in the database) are where they should be - right in the classes representing the entities. Which isn't that unique though - it's not original to PHP Jet and is similar to Doctrine, for example.
The basic philosophy is similar, but unlike the aforementioned Doctria, Jet DataModel is much smaller and simpler, solves many things much more easily, and there is a tool within Jet Studio where you can "snap" entities. For example, Jet DataModel doesn't need anything like Entity Manager, proxy classes and so on. Using DataModel is as straightforward, natural and simple as possible.
Example? Here they are:
/*
* Getting an article by ID
*/
$article = Content_Article::get( $id );
/*
* Change the date of the article:
*/
$article->setDateTime( Data_DateTime::now() );
$article->save();
/*
* Getting instances of all articles
*/
$articles = Content_Article::fetchInstances();
/*
* Retrieving instances of articles that are created a year ago and have the word PHP or Jet in their
* LOCALIZED headline
* using the INTERNAL RELATIONSHIP entity
*/
$articles = Content_Article::fetchInstances(
where: [
'date_time <=' => new Data_DateTime('-1 year'),
'AND',
'article_localized.title *' => ['PHP', 'Jet']
]
);
/*
* Pulling up a map of article titles in Czech localization. (Field key = article ID, field = title)
*/
$articles = Content_Article_Localized::dataFetchAssoc(
select: ['article_id', 'title'],
where: [
'locale' => new Locale('cs_CZ')
]
)
ORM DataModel is designed to achieve the best possible application performance. Thus, it offers various options to load data (partial loading, loading raw data, loading using different procedures, ...) and so on.
With a proper understanding of the ORM DataModel, it is possible to develop very powerful applications, portable to different database systems, while saving yourself a lot of routine and annoying work. Of course DataModel doesn't get in the way of your work and doesn't try to think for you!. It helps you with the routine and leaves the thinking to you - you know best what you are doing and why.
If you want, check out Jet Studio and create your own entity, or customize one of the ones the sample app already has. It's simple, absolutely simple.
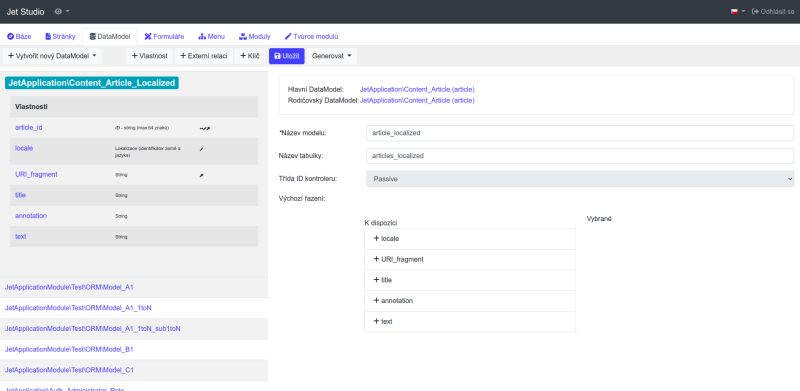
7. Configuration
You have already done the configuration by "clicking" in the web installer.
Jet has two levels of configuration. The basic configuration is the platform itself. This is used to set up things like enabling/disabling developer mode, caching, and a number of other things. Nothing special ...
You have filled out several forms in the installer. For example, you were creating a database connection, but not only that. And that's more interesting ;-)
PHP Jet has a application configuration system. The idea is that you can create configuration entities (classes with configuration definitions) and bind forms to them, and then very easily create, for example, that installer you went through.
8. Forms
And working with forms is of course not to be missed in PHP Jet. This is another very large topic, because the form support in PHP Jet is really very sophisticated - but still very simple in principle.
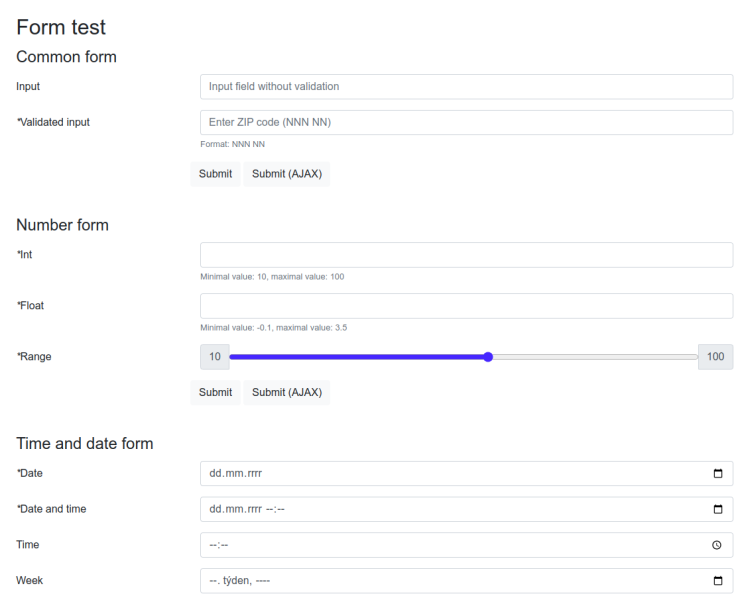
What does it do?
- Of course, the basic definition of the form and its fields using the appropriate classes.
- All sorts of field types are pre-implemented, but nothing prevents you from changing the implementation or creating new field types.
- Capturing input data (even from a REST request), validating data, handling errors, and securing input.
- Linking to the localization compiler - translation of everything from field labels to error messages.
- A system for easy, unified but still very flexible form rendering.
- Mapping forms directly to classes.
- A tool for clicking form definitions mapped to Jet Studio classes.
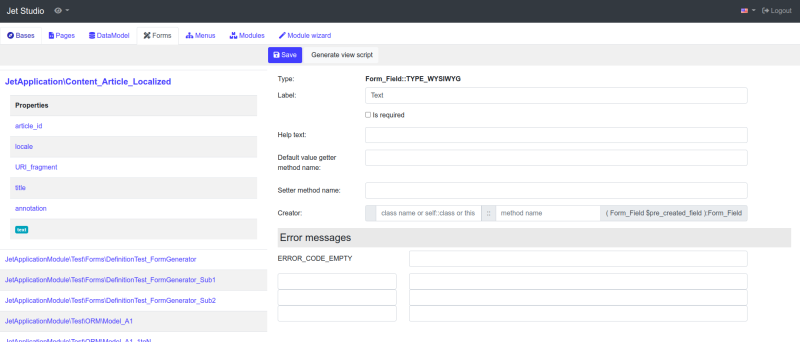
9. And so on...
And that's not all Jet can do... There's more:
- Thanks to the factories system, you have the ability to replace PHP Jet's "guts" with your own implementations without affecting the application from working. For example, are you uncomfortable with how the MVC page is implemented? There is nothing stopping you from creating your own implementation and simply embedding it into the system while keeping the whole philosophy and functionality.
- The authorization and authorization system is also very interesting.
- No developer should forget to use profiler.
- It also makes it easier to work with the application navigation.
- Jet also includes a system for generating UI on the server side and, of course, support for AJAX.
- And many other useful things like sending emails, working with images, working with tree structure of data, and so on, ...
If you have any questions, I've recently activated a discussion on GitHub, where I'll be happy to answer them, or you can still contact me directly.
Thanks for reading and I hope PHP Jet will make your work more enjoyable!